Prev Tutorial 3
Tutorial 4
Slider Control
Variables
GOAL: Experience
event-driven application with a Timer and Mouse events
PREREQUISITES: Tutorial
3
Add a Timer
- Add a label to echo the Timer, change
the label properties as:
A.
Name
to “Timer_Echo”
B.
Text
to “0”
C.
BorderStyle
to “Fixed3D
D.
AutoSize
to “False”
- Open
the ToolBox
- Above
“Common Controls” expand “All Window Forms”
- Drag a
Timer to the [Design] Window,
inside the properties window, change
A.
Enabled
to “true”
B.
Interval
to “1000”
4.
Create a Service Routine, again to service the Timer events
A. Go to Events
double-click on blank space to the right of Tick
5 Before
putting code into the Timer function block, go into Form1.Designer.cs
Add
in another private int variable, I called it counter.
6. Initialize
counter to “0” inside Form1.cs
7. Now
inside the function code block for timer1_Tick(...),
add the following code:
private void timer1_Tick(object sender, EventArgs e)
{
counter++;
Timer_Echo.Text
= counter.ToString() + “ : Seconds have passed”;
}
|
|
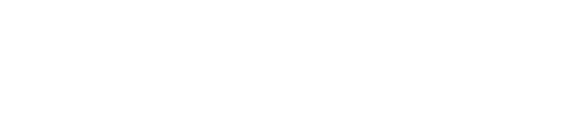
Create Mouse Echo
Text
- Add a
label to echo Mouse position,
change the label properties as:
A.
Name to
“Mouse_Echo”
B.
Text
to “”
C.
BorderStyle
to “Fixed3D”
D.
AutoSize
to “False”
Mouse Button Events
(Left and Right)
- Create
a Service Routine for MouseDown,
(This will create a routine that will trigger everytime a mouse button is
clicked, then within the routine you can decipher which mouse button it
was) to do this go to the [Design]
window
- Click
on the main window form, Form1,
go to Events
A.
Go to the Mouse
Events
B.
Double-Click on the blank space to the right of MouseDown
3.
Now inside the function code block for Form1_MouseDown(...), add the following
private void Form1_MouseDown(object sender, MouseEventArgs
e)
{
if (e.Button == MouseButtons.Left)
{
Mouse_Echo.Text
= “Left Mouse Down at “ + e.X.ToString() + “ , “ + e.Y.ToString();
}
if (e.Button == MouseButtons.Right)
{
Mouse_Echo.Text
= “Right Mouse Down at “ + e.X.ToString() + “ , “ + e.Y.ToString();
}
{
|
|
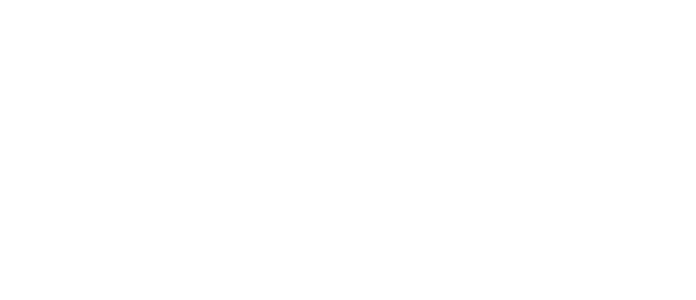
Mouse Move Event
- Create
a Service Routine for MouseMove,
to do this go to the [Design]
Window
- Click
on the main window form, Form1, go to Events
A. Go to the Mouse Events
B. Double-Click on the blank space
to the right of MouseMove
3. Now
inside the function code block for Form1_MouseMove(...),
add the following

§
In the Visual Studio project window, click
- Build
>> Build Solution
- Debug
>> Start to run
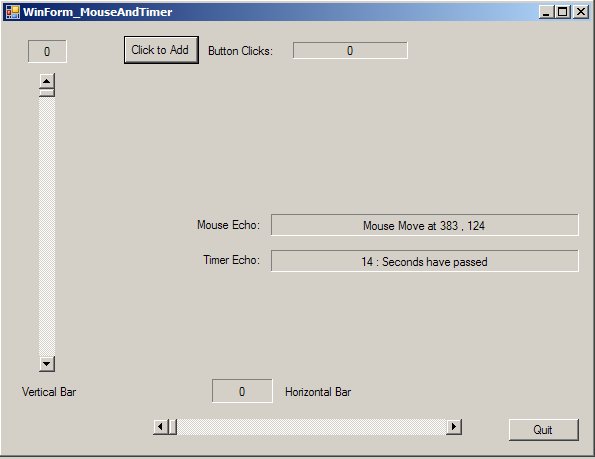
Next Tutorial 5